The following article contains affiliate links to Chatbase. If you end up signing up for a paid plan with Chatbase, I will get a commission. There's no additional cost to you.
Chatbase is a tool to simplify building a custom chatbot trained with whatever content you want. You can train it with content from files, raw text, or a website. Chatbase makes it trivial to train a chatbot and then embed it on your website.
For more technical users, Chatbase offers an API allowing you to create, train, and chat with Chatbase chatbots programmatically. This is invaluable for developers who don't want to maintain an integration with OpenAI, but are technical enough to integrate with Chatbase and customize the end user experience in ways that Chatbase doesn't support yet.
For example, you might want to customize the look and feel of a chatbot in ways Chatbase doesn't support. Or you might want to integrate Chatbase into other tools your organization uses such as support or internal company chat.
In this article, we'll go over what the API can do and cover some of its limitations. You'll even be able to chat with this very article.
API Access
The API is restricted to paying users of Chatbase. The lowest paid membership is $19/month and scale up from there offering more chatbots, messages/month, and characters/chatbot. Check out their pricing page for more information.
Once you've signed up for a subscription, navigate to your "Account" page and scroll to the bottom to create a new API key.
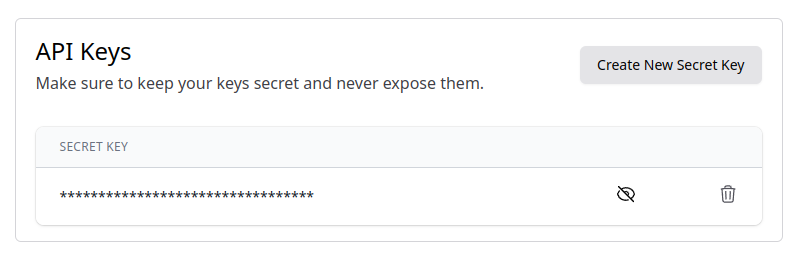
I suggest copying your API key and pasting it in your terminal as an environment variable to most easily follow along with this guide.
export CHATBASE_API_KEY=your_api_key_here
Otherwise, replace where I use $CHATBASE_API_KEY
with your API key in the sample API calls I include below.
Crawl a Website
Chatbase has an API to crawl a website and get all the site's URLs for you. This is a cool feature that they didn't really need to offer, but it's certainly nice to have. Using the response from this endpoint, you can drop in the list of links as source training data in the create chatbot call we'll look at next.
Note that for big websites the crawler may crash and not collect everything. In this case, you're better off creating your own crawler or referring to OpenAI's tutorial on creating a website Q&A that includes a website crawler and scraper.
You can also slim down the list of URLs included by passing a source URL to the API that is targeted on a particular path. For example, example.com/developers
to only get URLs within the /developers
part of a website.
This API is available through /api/v1/fetch-links
and accepts one URL parameter of sourceURL
for the URL of the website you want to scrape.
curl 'https://www.chatbase.co/api/v1/fetch-links?sourceURL=https://www.apimaestro.com' \
--request "GET" \
-H "Authorization: Bearer $CHATBASE_API_KEY"
The response body will contain an array called fetchedLinks
with objects containing a url
and size
. The size
field contains the number of characters present on the page. This is important to keep in mind because Chatbase limits how many characters you can train each chatbot on. The base $19/month plan is limited to 2 million characters per chatbot. The limit scales up along with the more premium plans. Refer to the Chatbase pricing page for more details.
The response body will also contain a stoppingReason
field indicating why the crawler may have stopped. According to Chatbase's docs, this most commonly happens when the crawler takes more than 60 seconds. The crawler will stop if it exceeds 60 seconds and will return only what it found in those 60 seconds. Their API docs don't currently indicate what else you might see in this field.
{
"fetchedLinks": [
{
"url": "https://www.apimaestro.com/",
"size": 4048
},
{
"url": "https://www.apimaestro.com/about/",
"size": 1405
},
{
"url": "https://www.apimaestro.com/what-is-openapi/",
"size": 6729
},
{
"url": "https://www.apimaestro.com/getting-started-with-pocket-bitcoins-api/",
"size": 15527
},
{
"url": "https://www.apimaestro.com/creating-an-openapi-specification-the-hard-way/",
"size": 18034
}
],
"stoppingReason": ""
}
Create a Chatbot
Now we'll create our first chatbot through the API. This is done making a POST request to the api/v1/create-chatbot
endpoint.
The request body should contain a chatbotName
and some source materials to train the bot. The source materials can be sent in 2 different fields:
urlsToScrape
to scrape text from web pages. This field should contain an array of URLs.sourceText
to use raw text to train the bot. As of my testing today, this field required at least 100 characters. The maximum number of characters depends on your plan.
You must provide at least one of the above fields. You can include both if you have a mix of URLs and raw text.
curl https://www.chatbase.co/api/v1/create-chatbot \
-H 'Content-Type: application/json' \
-H "Authorization: Bearer $CHATBASE_API_KEY" \
-d '{"sourceText": "risus sed vulputate odio ut enim blandit volutpat maecenas volutpat blandit aliquam etiam erat velit scelerisque in dictum non consectetur a erat nam at lectus urna duis convallis convallis tellus id interdum velit laoreet id donec ultrices tincidunt arcu non sodales neque sodales ut etiam sit amet nisl purus in", "urlsToScrape": ["https://apimaestro.com/getting-started-with-pocket-bitcoins-api/", "https://apimaestro.com/what-is-openapi/"], "chatbotName": "API Maestro"}'
The response body will contain a chatbotId
field with the ID of your chatbot.
{"chatbotId": "exampleId-123"}
You'll need this ID for all API calls chatting with or modifying your chatbot. If you fail to save the ID from the API response, you can always find it again in the Chatbase UI under your chatbot's settings page.
Possible Errors Creating a Chatbot
The Chatbase API docs don't explain anything about possible errors, but I was able to find a few playing around with this endpoint.
- If you don't pass any training material, you'll get a 500 error with message "Cannot read properties of undefined (reading 'replace')"
- If you're already at your limit for how many chatbots your plan allows, you'll get a 403 error with message "Limit to 5 chatbots for your plan. Delete existing chatbots or upgrade plan to increase the limit."
- If you pass too few characters as training material, you'll get a 403 error with message "Only 51 characters were extracted. undefined"
- If you pass too many characters as training material, you'll get a 403 with message "Exceeded character limit for your plan of 2000000 characters"
Updating a Chatbot
The Chatbase API also has an endpoint to update a chatbot via POST /api/v1/update-chatbot-data
. Currently, this endpoint only allows you to change the name of your chatbot and replace the training material. Whatever material you include in the update call will get rid of all the past training material you gave your bot
It's important to keep this replace function in mind because it means you must store on your side a list of all URLs and text you gave your chatbot and pass them again through this endpoint to ensure you don't tell your chatbot to forget anything. More on this in the challenges and limitations section of this guide.
This endpoint takes in all the same fields as the create call except you must also pass in a chatbotId
field.
curl https://www.chatbase.co/api/v1/update-chatbot-data \
-H 'Content-Type: application/json' \
-H "Authorization: Bearer $CHATBASE_API_KEY" \
-d '{"chatbotId": "exampleId-123", "sourceText": "Chatbase offers an API allowing you to create, train, and chat with Chatbase chatbots programmatically. This is invaluable for developers who don't want to maintain an integration with OpenAI, but are technical enough to integrate with Chatbase and customize the end user experience in ways that Chatbase doesn't support yet.", "chatbotName": "API Maestro new name"}'
The response body is the same as creating a chatbot. The possible errors are also the same from what I can tell.
Chatting with Your Chatbot
Finally, the moment you've been waiting for; chatting with your Chatbase chatbot. This is done through the POST /api/v1/chat
endpoint.
The request body should contain the following:
messages
which is an array containing each message in the chat. A message object contains arole
andcontent
. Supports up to a maximum of 7 messages.role
can beassistant
to refer to the chatbot anduser
to refer the human usercontent
is the message content from either the chatbot or human user
stream
which is a boolean where iftrue
will stream the chatbot's response to you word by word as raw text. Iffalse
, it will wait until the full response is generated before sending you the response as JSON.chatbotId
which is a string of your chatbot's ID
curl https://www.chatbase.co/api/v1/chat \
-H "Authorization: Bearer $CHATBASE_API_KEY" \
-d '{"messages":[{"content":"How can I help you?","role":"assistant"},{"content":"What is API Maestro?","role":"user"}],"chatId":"exampleId-123","stream":true}'
The response body, as mentioned previously, will be raw text with the chatbot's response if you set stream
as true. If you put stream
as false, you'll get the following JSON body back.
{
"text": "API Maestro helps API businesses grow by creating delightful developer experiences and documentation."
}
To continue chatting with the same context, you append the chatbot's last message to the messages
object and send another chat call. You can continue doing this until you have 7 messages in the messages
object according to their docs; although in practice I found I could go beyond 7 messages. This will probably be patched up eventually.
curl https://www.chatbase.co/api/v1/chat \
-H "Authorization: Bearer $CHATBASE_API_KEY" \
-d '{"messages":[{"content":"How can I help you?","role":"assistant"},{"content":"What is API Maestro?","role":"user"},{"content":"API Maestro helps API businesses grow by creating delightful developer experiences and documentation.","role":"assistant"},{"content":"Who runs this website?","role":"user"}],"chatId":"exampleId-123","stream":true}'
Challenges and Limitations
The Chatbase API has the basics down well, but it's very apparent the API is lacking in features compared to the UI experience.
For example, you can't set or modify anything for your chatbot except the name and training material through the API whereas the UI allows you to customize the prompt and GPT version.
You also can't see what training materials you've trained your chatbot with. Ideally, there would be a GET chatbot or GET chatbot training materials endpoint that would return the URLs and raw text you've used to train a bot.
Frustratingly, if you create a chatbot through the API, you also can't look up what you've trained it with through the UI either. It's all a black box whereas chatbots created through the UI will show you in the UI what source materials it's been trained with.
This introduces challenges particularly when updating a chatbot's training materials through the API. Unless you saved the list of URLs and text you sent to Chatbase when you created the chatbot, you run the risk of accidentally deleting old training material when you update your chatbot with new material.
With the lack of a GET chatbot endpoint, you also can't check in on your limits to see if you're near your cap of characters or messages.
There's a lot of promise here, but the API needs some work before it's ready for prime time. Nevertheless, it's amazing what Chatbase's creator Yasser has done by himself in just 2 months. I'm sure great things are still to come for both the UI and API experience.
What Next?
- Consider signing up for Chatbase.
- Check out the official docs.
- Follow the Chatbase founder's Twitter for product updates and follow how his business is growing.
- Try chatting with this article: